showAuth
client.showAuth({ container: HTMLElement|id, auth: object, // Optional arguments allowLogin: boolean, allowWebAuthnLogin: boolean, allowSignup: boolean, allowWebAuthnSignup: boolean, allowForgotPassword: boolean, allowPhoneNumberResetPassword: boolean, allowAccountRecovery: boolean, allowCustomIdentifier: boolean, allowAuthentMailPhone: boolean, allowTrustDevice: boolean, enablePasswordAuthentication: boolean, initialScreen: string, signupFields: string[]|object[], (1) redirectUrl: string, socialProviders: string[], showLabels: boolean, showRememberMe: boolean, canShowPassword: boolean, displaySafeErrorMessage: boolean, countryCode: string, onReady: function, onSuccess: function, onError: function, returnToAfterEmailConfirmation: string, (2) returnToAfterPasswordReset: string, (2) theme: object, i18n: object, recaptcha_enabled: boolean, recaptcha_site_key: string, phoneNumberOptions: object })
1 | You can force certain fields to be optional when needed by passing the key for the field and required: false as shown in the Examples. |
2 | These parameters should only be used with Hosted Pages. |
Description
Show the authentication widget with signup, login, and forgot password forms.
|
Examples
// The SMS feature is disabled on the ReachFive account
client.showAuth({
container: 'auth-container',
auth: {
redirectUri: 'https://example.com/auth-callback'
},
allowForgotPassword: false,
allowPhoneNumberResetPassword: false,
allowAuthentMailPhone: true,
initialScreen: 'login',
redirectUrl: 'https://example-email-validate.com',
signupFields: [
{ key: 'given_name', label: 'Given name', required: false },
{ key: 'family_name', label: 'Family name', required: false },
'email',
'password',
'password_confirmation',
'consents.newsletter',
'custom_fields.loyalty_card_number'
],
socialProviders: ['facebook', 'google'],
showLabels: true,
showRememberMe: true,
countryCode: 'US',
onReady: instance => {
// Destroy the widget
// if (...) instance.destroy()
},
onSuccess: () => {
console.log("Success!")
},
onError: (error: ErrorResponse) => {
console.error(error)
},
theme: {
primaryColor: '#274890',
borderRadius: '25',
socialButton: {
inline: true
}
},
i18n: {
email: 'Email'
}
})
Parameters
Boolean that specifies if the forgot password option is enabled.
|
|||||||||||||||||||||||||||||||||
Boolean that specifies whether quick login is enabled. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether password authentication is enabled or not. Defaults to
|
|||||||||||||||||||||||||||||||||
Boolean that specifies whether biometric login is enabled. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether biometric signup is enabled. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether login is enabled. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether signup is enabled. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether an additional field for the custom identifier is shown. Defaults to |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether to show the option to authenticate via email or phone number.
Defaults to |
|||||||||||||||||||||||||||||||||
List of authentication options
|
|||||||||||||||||||||||||||||||||
Whether or not to provide the display password in clear text option. Defaults to |
|||||||||||||||||||||||||||||||||
The DOM element or the |
|||||||||||||||||||||||||||||||||
The ISO country code useful to format phone numbers. Defaults to the predefined country code in your account settings or |
|||||||||||||||||||||||||||||||||
Whether or not to display a safe error message on password reset, given an invalid email address. This mode ensures not to leak email addresses registered to the platform. Defaults to |
|||||||||||||||||||||||||||||||||
Widget labels and error messages to override.
Falls back to the default wordings in Using the For example, you can use the Example
|
|||||||||||||||||||||||||||||||||
The widget’s initial screen. See |
|||||||||||||||||||||||||||||||||
Callback function called when the request has failed. See our full list of SDK and API errors. You can override this function with custom logic if desired: client.showAuth example
|
|||||||||||||||||||||||||||||||||
Callback function called after the widget has been successfully loaded and rendered inside the container. The callback is called with the widget instance. |
|||||||||||||||||||||||||||||||||
Callback function called when the request was successful. You can override this function with custom logic if desired: client.showAuth example
|
|||||||||||||||||||||||||||||||||
Boolean if the account recovery option is displayed or not. Defaults to
|
|||||||||||||||||||||||||||||||||
The URL sent in the email to which the user is redirected. This URL must be whitelisted in the |
|||||||||||||||||||||||||||||||||
Returned in the
|
|||||||||||||||||||||||||||||||||
Returned in the
|
|||||||||||||||||||||||||||||||||
Boolean for whether the signup form fields' labels are displayed on the login view. Defaults to |
|||||||||||||||||||||||||||||||||
Whether the Remember me checkbox is displayed on the login view. Affects user session duration. The account session duration configured in the ReachFive Console ( ) applies when:
If the checkbox is visible and not selected by the user, the default session duration of 1 day applies. Defaults to |
|||||||||||||||||||||||||||||||||
List of the signup fields to display in the form.
A field is either a You can pass a field as an object to override default values:
|
|||||||||||||||||||||||||||||||||
Lists the available social providers. This is an array of strings. If you pass an empty array, social providers are not displayed. If You can also specify variants for a provider as a key:value pair:
|
|||||||||||||||||||||||||||||||||
The options to set up to customize the appearance of the widget. Below is an example of some of the theme attributes. For a full list, see theme attributes.
|
|||||||||||||||||||||||||||||||||
Boolean that specifies whether reCAPTCHA is enabled or not. |
|||||||||||||||||||||||||||||||||
Object that lets you set display options for the phone number field. Options
|
|||||||||||||||||||||||||||||||||
Boolean that specifies whether to display the option to trust the device. values
Defaults to |
|||||||||||||||||||||||||||||||||
The SITE key that comes from your reCAPTCHA setup. This must be paired with the appropriate secret key that you received when setting up reCAPTCHA. |
|||||||||||||||||||||||||||||||||
Boolean that specifies whether or not users can use a phone number to reset their password. values
Defaults to |
initialScreen
logic
The initialScreen
parameter sets the widget’s initial screen.
Depending on your requirements, this can be explicitly set to a certain screen.
The options are:
Value | Description |
---|---|
|
Displays the login screen. |
|
Displays the login screen with WebAuthn options such as biometrics. |
|
Displays the signup screen. |
|
Displays the forgot-password screen. |
However, if no value is provided, the initial screen follows the logic provided here.
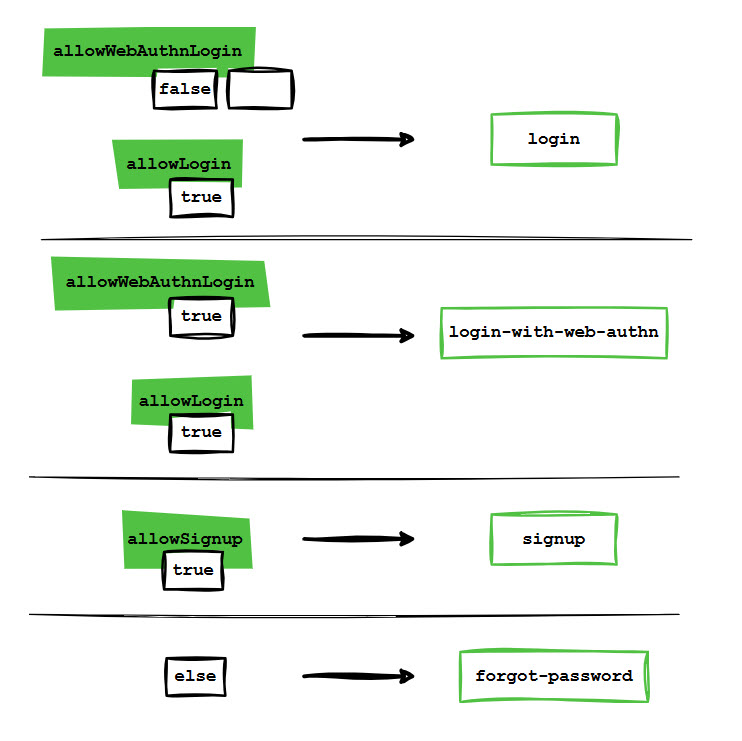
theme
attributes
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
UI labels
ReachFive has a set of default labels for UI components and fields. This includes a range of things from names and sign up fields to verification code fields, and more.
Using the i18n
field, you can override these default labels to suit your particular setup.
client.showAuth({
i18n: {
email: 'Your email address, por favor.'
},
…
})