webviewLogin
AppDelegate.reachfive().webviewLogin(WebviewLoginRequest( presentationContextProvider // optional state, nonce, scope, (1) origin, prefersEphemeralWebBrowserSession, (2) ))
1 | Scope isn’t explicitly required. If not provided here, it defaults to the scopes set up in the client configuration which is picked up when you initialize the iOS SDK. |
2 | Available starting with version 7.1.3 |
Description
Opens a secure webview through the authorization endpoint.
You must have configured a Login URL and enabled request orchestration tokens for your identity client. Orchestrated flows are supported from version |
-
If end-users have an active web SSO session, then the authorization endpoint immediately redirects to the application.
-
If end-users do not have an active web SSO session, they are redirected to the client’s Login URL for authentication.
When logging in with secure webview, a dialog pops up where you must select Continue and acknowledge that by continuing "This allows the app and the website to exchange information about you".
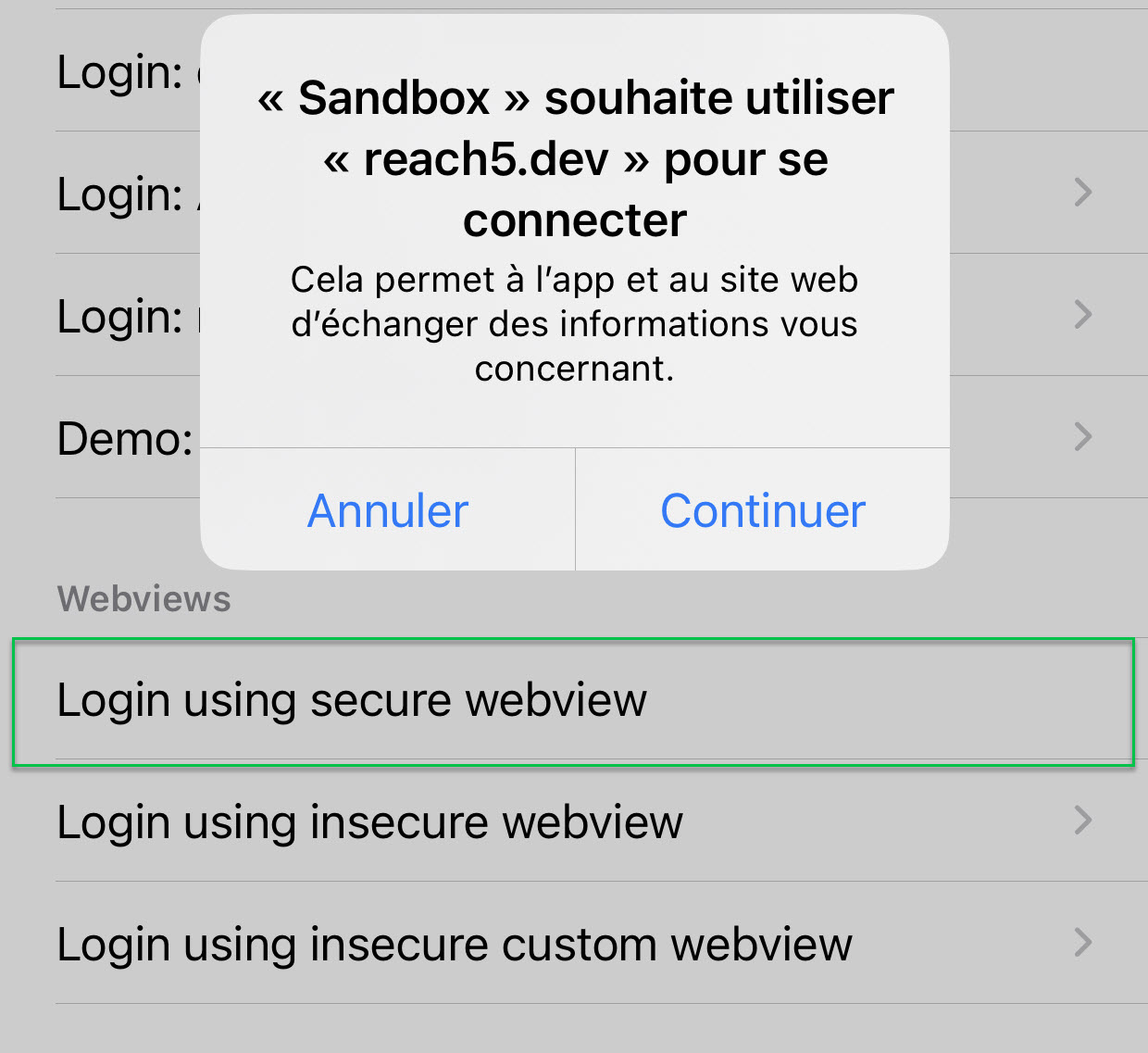
Usage
Because of the presentationContextProvider
property, you must ensure that your UIViewController implements the ASWebAuthenticationPresentationContextProviding
protocol.
To do this, add the following to your UIViewController:
func presentationAnchor(for session: ASWebAuthenticationSession) -> ASPresentationAnchor {
view.window!
}
Examples
AppDelegate
.reachfive()
.webviewLogin(WebviewLoginRequest(
state: "zf3ifjfmdkj",
nonce: "n-0S6_PzA3Ze",
scope: ["openid", "profile", "email"],
presentationContextProvider: self
))
.onSuccess{ authToken in
// Get the profile's authentication token
}
.onFailure { error in
// Return a ReachFive error
}
Parameters
Parameter | Description | ||
---|---|---|---|
A delegate that provides a display context whereby the system is able to present an authentication session to the user.
|
|||
The OAuth2 state value.
|
|||
An OIDC nonce value.
|
|||
The scopes granted to the profile. Make sure they are allowed by the client. Default scopes are the allowed scopes set up in the client’s configuration. |
|||
The origin of the call. |
|||
A Boolean value that indicates whether the session should ask the browser for a private authentication session. The value of this property is false by default.
|
Response
Type: Future<AuthToken, ReachFiveError>
AuthToken
The authentication token.
idToken |
The ID token JSON Web Token (JWT) that contains the profile’s information. This is only available when the |
||||||||||||||||||||||||||||||||||
accessToken |
The authorization credential JSON Web Token (JWT) used to access the ReachFive API. |
||||||||||||||||||||||||||||||||||
refreshToken |
The refresh token JSON Web Token (JWT) used to obtain new access tokens once they expire. This is only available when the |
||||||||||||||||||||||||||||||||||
tokenType |
The type of token. Always equal to |
||||||||||||||||||||||||||||||||||
expiresIn |
The lifetime in seconds of the access token. If |
||||||||||||||||||||||||||||||||||
user OpenIDUser |
The user’s information contained in the ID token.
|
ReachFiveError
Based on the problem, the ReachFiveError
will be:
-
AuthCanceled
: The user cancelled the request or no credential was available in the keychain. -
RequestError(apiError: ApiError)
for a Bad Request (status 400) error. -
AuthFailure(reason: String, apiError: ApiError?)
mainly for Unauthorized (status 401) error. -
TechnicalError(reason: String, apiError: ApiError?)
if it’s an Internal Server Error (status 500) or other internal errors.
ApiError
error |
The main error message. |
||||||
errorId |
The identifier of the error. |
||||||
errorUserMsg |
The user-friendly error message.
|
||||||
errorMessageKey |
The error message key. |
||||||
errorDescription |
The technical error message. |
||||||
errorDetails FieldError[] |
|