Account recovery
You can initiate account recovery with the requestAccountRecovery or requestPasswordReset method, depending on your implementation of our SDK. In any case, account recovery is a multi-step account recovery process, which enables your users to regain access to their accounts by updating their password or resetting passkeys.
This guide outlines the steps required to implement the full flow, from triggering the recovery request to completing the action with a verification code.
Integrators must handle each step, including optional callback mechanisms, to ensure a seamless experience.
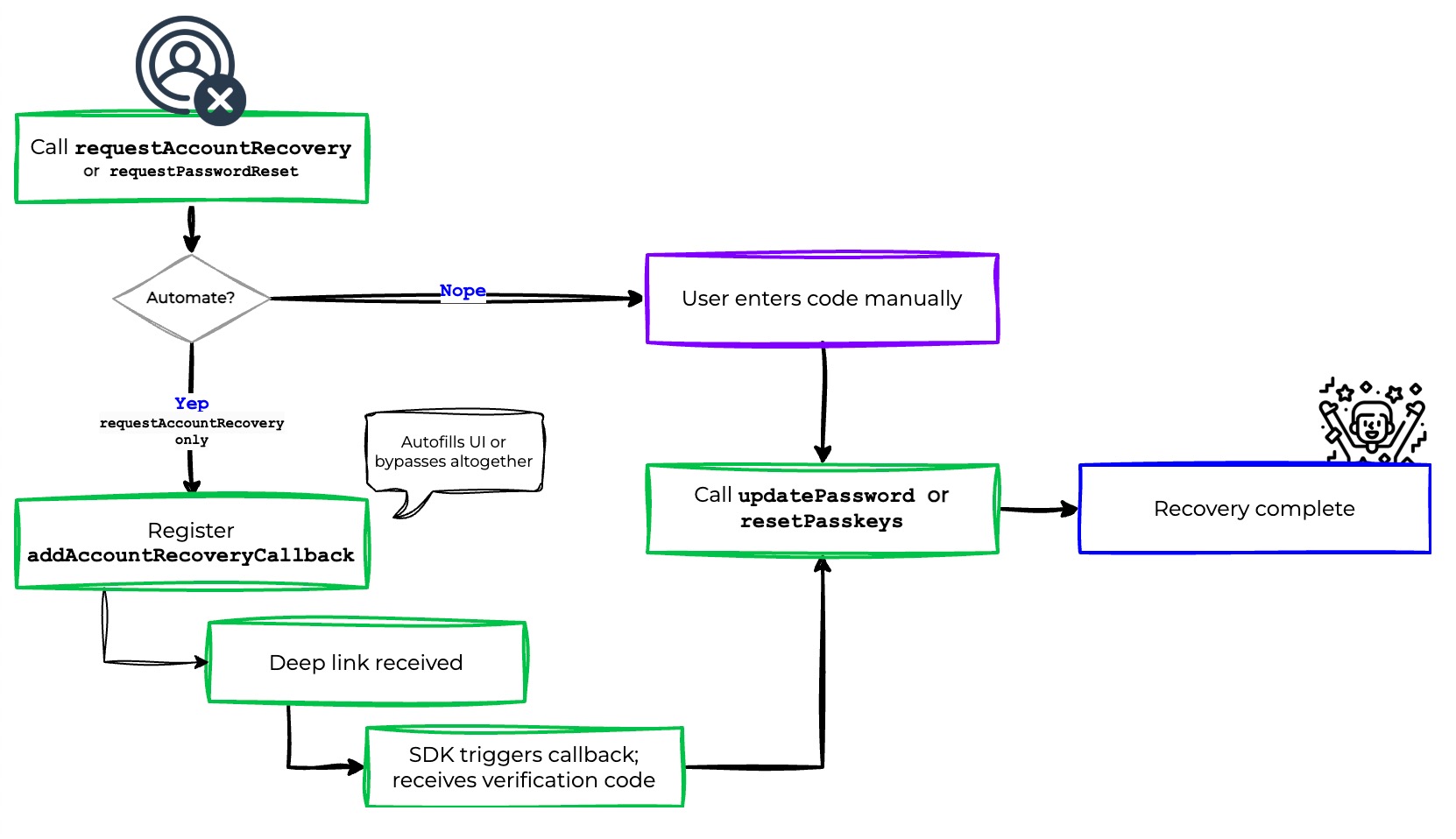
Initiate the recovery request
First, you need to:
-
Call requestAccountRecovery or requestPasswordReset with the user’s identifier (e.g., email or phone number).
-
Our SDK sends a verification code to the user via the configured channel (e.g., email/SMS).
Email exampleAppDelegate .reachfive() .requestAccountRecovery( email: "john.doe@gmail.com", redirectUrl: "reachfive-clientId://account-recovery", ) .onSuccess { _ in // Do something } .onFailure { error in // Return a ReachFive error }
Email exampleAppDelegate .reachfive() .requestPasswordReset( email: "john.doe@gmail.com", redirectUrl: "reachfive-clientId://password-reset" ) .onSuccess { _ in // Do something } .onFailure { error in // Return a ReachFive error }
Capture the verification code
Optionally, implement addAccountRecoveryCallback
to automatically receive the verification code via deep link.
You should provide a UI for manual verification code entry if callback isn’t used.
addAccountRecoveryCallback
will autofill or bypass the UI in this case though.
You should store the code securely to complete the recovery process.
addAccountRecoveryCallback
addAccountRecoveryCallback
only works when requestAccountRecovery
is used, not with requestPasswordReset
.
addAccountRecoveryCallback
allows you to register a closure that automatically receives the verification code.
This is typically delivered via a deep link after the user interacts with the recovery email or SMS.
This optional approach eliminates the need for manual code entry by capturing the code directly in the app. Once set, the closure is triggered by our iOS SDK with the verification code, which can then be passed to updatePassword or resetPasskeys to complete the recovery process.
Refer to addAccountRecoveryCallback for further details.
Complete the recovery process
To complete the recovery process, use the verification code to finalize recovery:
-
Call updatePassword with the code and new password to update the user’s password.
This is common for account recovery or password reset flows.
-
Alternatively, call resetPasskeys with the code to reset passkeys (specific to account recovery).
-
Be sure to handle both success (e.g., “Recovery complete”) and errors (e.g., invalid/expired code).