Getting Started
What’s new in 7.0.3?
All properties are now nullable. We have also suppressed some ProGuard rules.
Requirements
The ReachFive SDK supports Android SDK from API Level 19 (Android version 4.4
KitKat).
To initialize the ReachFive client:
-
You need a Domain URL and Client ID.
-
You must whitelist all available domains where the ReachFive SDK will be used.
This is done in the Allowed Origins (CORS) field of your ReachFive console, in the Settings menu.
Installation
ReachFive SDKs are published to Maven Central as separate modules.
allprojects {
repositories {
mavenCentral() (1)
}
}
1 | Add mavenCentral as a repository location in your project’s top-level build.gradle file. |
Below are installation instructions for each module. |
SDK Core
Core contains all the main tools and interfaces, and the methods related to standard authentication with an identifier and a password.
Add the following line to your app/build.gradle
, replacing x.y.z
with the latest SDK version:
dependencies {
implementation "co.reachfive.identity:sdk-core:x.y.z"
}
The following permissions are required to communicate with the ReachFive servers. Add them to the AndroidManifest.xml
file.
<manifest>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.USE_CREDENTIALS" />
</manifest>
SDK WebView
This module uses a WebView to authenticate users, it enables all providers that are supported by ReachFive.
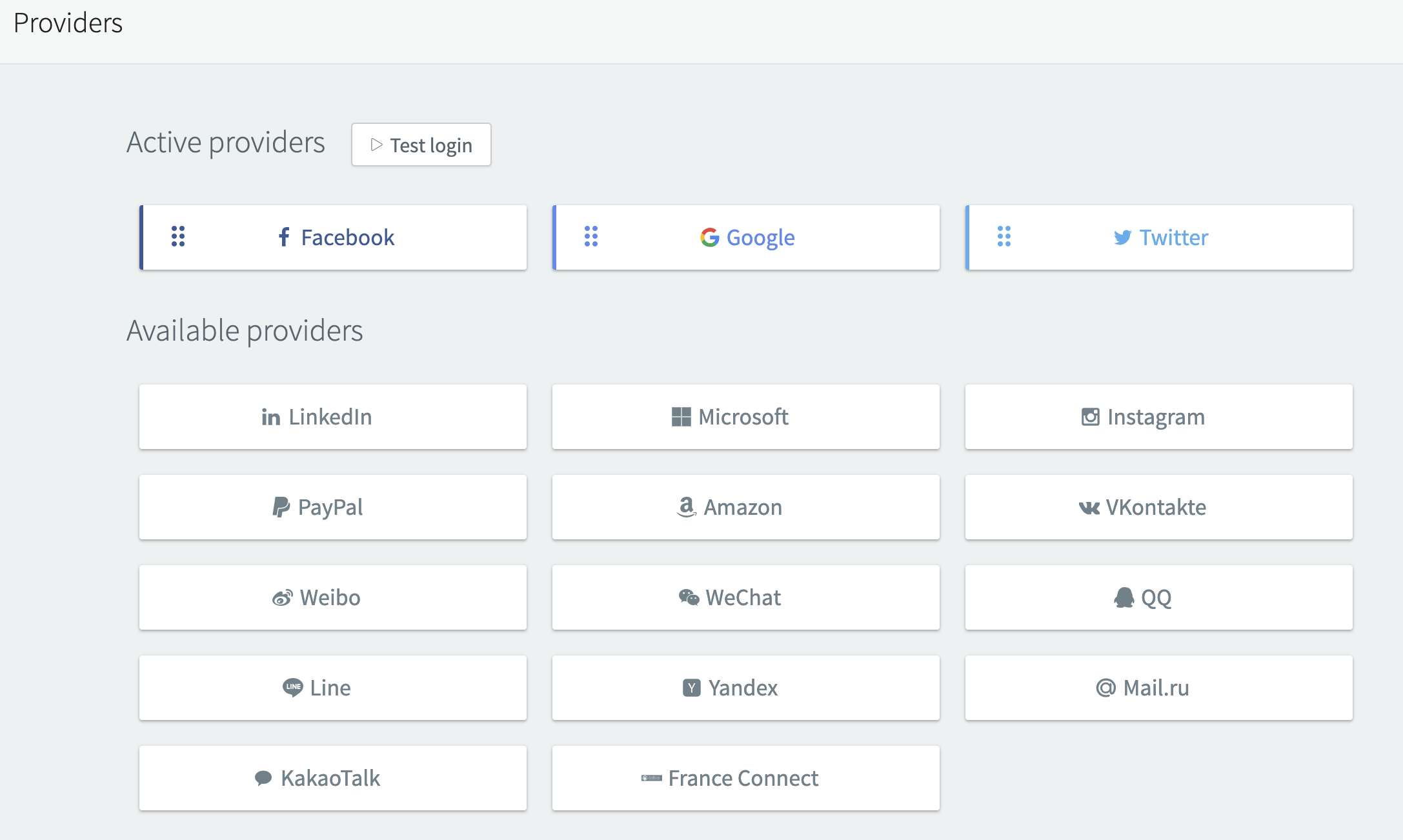
Add the following line to your app/build.gradle
, replacing x.y.z
with the latest version:
dependencies {
implementation "co.reachfive.identity:sdk-webview:x.y.z"
}
You need to add this activity into the AndroidManifest.xml
file:
<activity
android:name="co.reachfive.identity.sdk.webview.ReachFiveLoginActivity"
android:screenOrientation="portrait" />
For mobile contexts, you must whitelist This URL scheme is the default callback URL and is used to request a token when implementing the SDK Webview. |
Facebook native provider
This module uses the Facebook native SDK to provide a better user experience.
Add the following lines to your app/build.gradle
, replacing x.y.z
with the latest version:
dependencies {
implementation "co.reachfive.identity:sdk-facebook:x.y.z"
implementation "com.facebook.android:facebook-login:12.2.0"
}
Refer to the Meta Connect (Facebook Login) guide to create your Facebook application.
If you’re using the latest version of the Facebook API, please remove the user_gender scope from the ReachFive client configuration to prevent any issues.
|
Add the line below into your string.xml
resource file with your Facebook application ID:
<resources>
<string name="facebook_app_id">FACEBOOK_APPLICATION_ID</string>
</resources>
Then add these lines into the AndroidManifest.xml
file:
<manifest>
<meta-data
android:name="com.facebook.sdk.ApplicationId"
android:value="@string/facebook_app_id" />
<activity
android:name="com.facebook.FacebookActivity"
android:configChanges="keyboard|keyboardHidden|screenLayout|screenSize|orientation"
android:label="@string/app_name" />
</manifest>
Configure Google native provider
This module uses the Google native SDK to provide a better user experience.
-
First, include Google’s Maven repository in your top-level
build.gradle
file:allprojects { repositories { google() } }
To enable the service, add the line below at the bottom of your
|
-
Add the following line to your
app/build.gradle
, replacingx.y.z
with the latest version:dependencies { implementation "co.reachfive.identity:sdk-google:x.y.z" }
-
Use the Google Connect guide to create your Google application.
-
Create a new Firebase project.
-
Once the project is created, configure the release (
keystore.jks
) and production (Google Play) SHA1s in FireBase with the application’s package name as shown with the command below:keytool -list -v -keystore [keystorePath] - alias [aliasName] -storepass [storePassword] -keypass [keyPassword]
More information about the keytool utility in the Authenticating Your Client guide. |
The ANDROID AUTH2 CLIENTS are generated automatically in the Google Console API through Firebase. |
-
Download the
google-services.json
file and put it at the root of your Android project. You can find more information in Add Firebase to your Android project and Adding the JSON File.
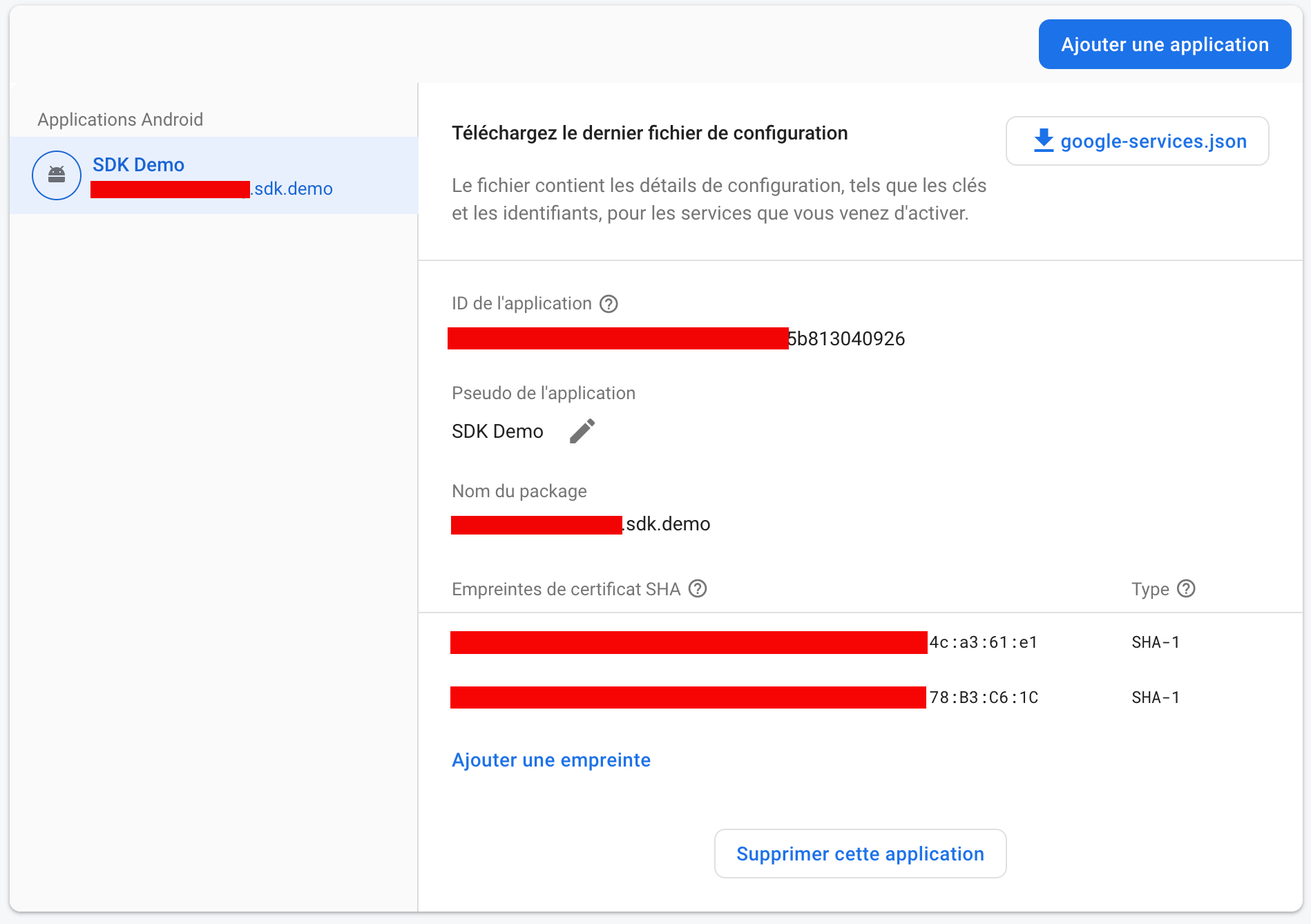

Initialization
Below are all the functions accessible via the Android SDK.
In all the code examples below, the ReachFive client is instantiated and stored in a variable named client
.
import android.content.Intent
import android.os.Bundle
import android.util.Log
import android.view.Menu
import android.view.MenuItem
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import co.reachfive.identity.sdk.core.ReachFive
import co.reachfive.identity.sdk.core.SdkConfig
import co.reachfive.identity.sdk.core.models.AuthToken
import co.reachfive.identity.sdk.core.models.ProfileSignupRequest
import co.reachfive.identity.sdk.facebook.FacebookProvider
import co.reachfive.identity.sdk.google.GoogleProvider
import co.reachfive.identity.sdk.webview.WebViewProvider
import io.github.cdimascio.dotenv.dotenv
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
// The ReachFive client
private lateinit var client: ReachFive
val DOMAIN = "Your ReachFive domain"
val CLIENT_ID = "Your ReachFive client ID"
val SCHEME = "Your ReachFive scheme" (1)
override fun onCreate(savedInstanceState: Bundle?) {
// The configuration parameters needed by the ReachFive client
val sdkConfig = SdkConfig(domain = DOMAIN, clientId = CLIENT_ID, scheme = SCHEME)
// The list of the social providers needed by your application
val providersCreators = listOf(GoogleProvider(), FacebookProvider(), WebViewProvider())
client = ReachFive(
sdkConfig = sdkConfig,
providersCreators = providersCreators,
// Your Android activity
activity = this
)
// Initialize the ReachFive client
client.initialize({ providers ->
// On success, do something with the retrieved list of providers registered for this ReachFive client
// ...
}, {
// On failure, log the error message returned by the ReachFive client
Log.d("Reach5_MainActivity", "ReachFive init ${it.message}")
})
}
}
1 | The SCHEME should follow the format: reachfive://${clientId}/callback . It is a URI for native apps. |